One of the most common object in Windows Phone application is bitmap.
The image element displays bitmap.
Most popular bitmap format are :
- JPEG
- PNG
- GIF
Note that Silverlight supports only JPEG and PNG !!
The image class
Image element in XAML is decribed by <Image .../> in general <Image Source="uri"/> where source is the URI of the bitmap file.
URI is a representation of a resource on the internet or intranet.
I will demonstrate two use case regarding the resource location :
- Bitmap reside localy
- Bitmap reside on the internet
Local bitmap
Add directory to the project
Give the new directory a name - Image.
Next add to Image directory bitmap file name LocalBitmap.jpg
A directory name Image was created at the same level as the project file and a LocalBitmap.jpg reside inside it.
The project looks now like this :
Now mark LocalBitmap.png, right click mouse, choose properties and change "Build Action" to Content and "Copy to Output Directory" to "Copy if newer".
Now after compilation this will copy LocalBitmap.jpg to the directory Image which reside on the same directory as the xap file
Add to the MainPag.xaml the image element which point locally to the bitmap file
Run the application to get
LocalBitmap.zip
Internet Bitmap
Here the bitmap file reside somwhere on the internet.
So simple add the following image element.
Run the application and the result is :
InternetBitmap.zip
Dynamic bitmap load
The samples before handled static loading of bitmap i.e. the bitmap file path was written in the XAML and was known before the application was loaded. It was handled very well by Image.Source type ImageSource which is abstract class. If you think a little about these two samples you must admire the work done here. By simply adding a path into an Image element on the XAML you get a display of local bitmap file in one sample and a display of a bitmap file which reside on the internet (and naturally involve network API to access it) on a second sample.
When you need to do this dynamically it looks like this :
First i add two image elements to MainPage.xaml
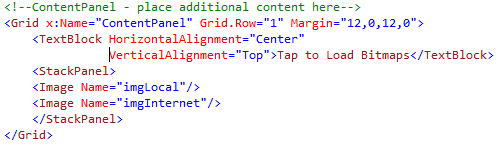
Then i use BitmapSource which inherits ImageSource to load the bitmaps.
Bitmap loading is activated after a tap and handled by the static touch handler - this was covered in previous post (Touch of Touch (3))
DynamicLoadBitmap.zip
Nathan